Go参考手册
编码 | encoding
encoding/asn1
- import "encoding/asn1"
- 概述
- 索引
概述
按照 ITU-T Rec X.690 的规定,asn1 包实现了对 DER 编码的 ASN.1 数据结构的解析。
另见“ ASN.1,BER 和 DER 子集的外行指南”,http://luca.ntop.org/Teaching/Appunti/asn1.html。
索引
- 常量
- 变量
- func Marshal(val interface{}) ([]byte, error)
- func Unmarshal(b []byte, val interface{}) (rest []byte, err error)
- func UnmarshalWithParams(b []byte, val interface{}, params string) (rest []byte, err error)
- type BitString
- func (b BitString) At(i int) int
- func (b BitString) RightAlign() []byte
- type Enumerated
- type Flag
- type ObjectIdentifier
- func (oi ObjectIdentifier) Equal(other ObjectIdentifier) bool
- func (oi ObjectIdentifier) String() string
- type RawContent
- type RawValue
- type StructuralError
- func (e StructuralError) Error() string
- type SyntaxError
- func (e SyntaxError) Error() string
包文件
asn1.go common.go marshal.go
常量
ASN.1 标签表示以下对象的类型。
const (
TagBoolean = 1
TagInteger = 2
TagBitString = 3
TagOctetString = 4
TagNull = 5
TagOID = 6
TagEnum = 10
TagUTF8String = 12
TagSequence = 16
TagSet = 17
TagPrintableString = 19
TagT61String = 20
TagIA5String = 22
TagUTCTime = 23
TagGeneralizedTime = 24
TagGeneralString = 27
)
ASN.1 类类型表示标签的名称空间。
const (
ClassUniversal = 0
ClassApplication = 1
ClassContextSpecific = 2
ClassPrivate = 3
)
变量
NullBytes 包含表示 DER 编码的 ASN.1 NULL 类型的字节。
var NullBytes = []byte{TagNull, 0}
NullRawValue 是一个 RawValue,其标签设置为 ASN.1 NULL 类型 tag(5)。
var NullRawValue = RawValue{Tag: TagNull}
func Marshal(查看源代码)
func Marshal(val interface{}) ([]byte, error)
Marshal 返回 val 的 ASN.1 编码。
除了由 Unmarshal 识别的结构标签之外,还可以使用以下内容:
ia5:导致字符串被封送为ASN.1,IA5String值
omitempty:导致跳过空切片
printable:导致字符串被封送为ASN.1,PrintableString值
utf8:导致字符串被封送为ASN.1,UTF8String值
utc:导致time.Time被封送为ASN.1,UTCTime值
generalized:导致time.Time被封送为ASN.1,GeneralizedTime值
func Unmarshal(查看源代码)
func Unmarshal(b []byte, val interface{}) (rest []byte, err error)
Unmarshal 分析 DER 编码的 ASN.1 数据结构 b 并使用反射包填充 val 指向的任意值。由于 Unmarshal 使用反射包,因此写入的结构必须使用大写字段名称。
ASN.1 INTEGER 可以写入 int,int32,int64 或 *big.Int(来自 math/big package)。如果编码值不适合 Go 类型,Unmarshal 会返回解析错误。
一个 ASN.1 BIT STRING 可以写入一个 BitString。
ASN.1 OCTET STRING 可以写入 []byte。
一个 ASN.1 OBJECT IDENTIFIER 可以写入一个 ObjectIdentifier。
一个 ASN.1 枚举可以被写入枚举。
一次 ASN.1 UTCTIME 或 GENERALIZEDTIME 可写入一次。
ASN.1 PrintableString 或 IA5String 可写入字符串。
任何上面的 ASN.1 值都可以写入 interface{}。存储在接口中的值具有相应的 Go 类型。对于整数,该类型是 int64。
如果可以将 x 写入片段的元素类型,则可以将 x 或 x 的 ASN.1 序列写入片段。
如果可以将序列中的每个元素写入结构中的相应元素,则可以将 ASN.1 SEQUENCE 或 SET 写入结构。
结构字段上的以下标记对 Unmarshal 具有特殊意义:
application指定使用APPLICATION标记
default:x设置可选整数字段的默认值(仅在可选的整数字段存在时使用)
explicit指定附加的显式标记包装隐式标记
可选标记该字段为ASN.1可选
set会导致SET,而不是SEQUENCE类型
tag:x指定ASN.1标记号; 暗示ASN.1特定背景
如果结构的第一个字段的类型是 RawContent,那么该结构的原始 ASN1 内容将被存储在其中。
如果一个切片元素的类型名称以“SET”结尾,那么它将被视为设置了“set”标签。这可以用于无法给出 struct 标记的嵌套切片。
其他 ASN.1 类型不受支持;如果遇到,Unmarshal 将返回一个分析错误。
func UnmarshalWithParams(查看源代码)
func UnmarshalWithParams(b []byte, val interface{}, params string) (rest []byte, err error)
UnmarshalWithParams 允许为顶层元素指定字段参数。参数的形式与字段标签相同。
type BitString(查看源代码)
当您需要 ASN.1 BIT STRING 类型时,BitString 是要使用的结构。位串被填充到内存中最近的字节并记录有效位的数量。填充位将为零。
type BitString struct {
Bytes []byte // 比特打包成字节。
BitLength int // 比特长度。
}
func (BitString) At(查看源代码)
func (b BitString) At(i int) int
返回给定索引处的位。如果索引超出范围,则返回 false。
func (BitString) RightAlign(查看源代码)
func (b BitString) RightAlign() []byte
RightAlign 返回填充位在开始位置的片。切片可以与 BitString 共享内存。
type Enumerated(查看源代码)
Enumerated 被表示为一个纯(plain) int。
type Enumerated int
type Flag(查看源代码)
Flag 接受任何数据,如果存在则设置为 true。
type Flag bool
type ObjectIdentifier(查看源代码)
一个 ObjectIdentifier 表示一个 ASN.1 对象标识符。
type ObjectIdentifier []int
func (ObjectIdentifier) Equal(查看源代码)
func (oi ObjectIdentifier) Equal(other ObjectIdentifier) bool
平等报告 oi 和其他是否表示相同的标识符。
func (ObjectIdentifier) String(查看源代码)
func (oi ObjectIdentifier) String() string
type RawContent(查看源代码)
RawContent 用于表示未解码的 DER 数据需要为结构体保留。要使用它,结构的第一个字段必须有这种类型。任何其他字段都有这种类型的错误。
type RawContent []byte
type RawValue(查看源代码)
一个 RawValue 表示一个未解码的 ASN.1 对象。
type RawValue struct {
Class, Tag int
IsCompound bool
Bytes []byte
FullBytes []byte // 包括标签和长度
}
type StructuralError(查看源代码)
StructuralError 表明 ASN.1 数据是有效的,但是接收它的 Go 类型不匹配。
type StructuralError struct {
Msg string
}
func (StructuralError) Error(查看源代码)
func (e StructuralError) Error() string
type SyntaxError(查看源代码)
SyntaxError 表明 ASN.1 数据无效。
type SyntaxError struct {
Msg string
}
func (SyntaxError) Error(查看源代码)
func (e SyntaxError) Error() string
编码 | encoding相关
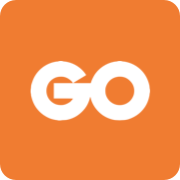
Go 是一种编译型语言,它结合了解释型语言的游刃有余,动态类型语言的开发效率,以及静态类型的安全性。它也打算成为现代的,支持网络与多核计算的语言。要满足这些目标,需要解决一些语言上的问题:一个富有表达能力但轻量级的类型系统,并发与垃圾回收机制,严格的依赖规范等等。这些无法通过库或工具解决好,因此Go也就应运而生了。
主页 | https://golang.org/ |
源码 | https://go.googlesource.com/go |
发布版本 | 1.9.2 |