Go参考手册
编码 | encoding
encoding/xml
- import "encoding/xml"
- 概述
- 索引
- 示例
概述
Package xml 实现了一个理解 XML 名称空间的简单 XML 1.0 分析器。
索引
- 常量
- 变量
- func Escape(w io.Writer, s []byte)
- func EscapeText(w io.Writer, s []byte) error
- func Marshal(v interface{}) ([]byte, error)
- func MarshalIndent(v interface{}, prefix, indent string) ([]byte, error)
- func Unmarshal(data []byte, v interface{}) error
- type Attr
- type CharData
- func (c CharData) Copy() CharData
- type Comment
- func (c Comment) Copy() Comment
- type Decoder
- func NewDecoder(r io.Reader) *Decoder
- func (d *Decoder) Decode(v interface{}) error
- func (d *Decoder) DecodeElement(v interface{}, start *StartElement) error
- func (d *Decoder) InputOffset() int64
- func (d *Decoder) RawToken() (Token, error)
- func (d *Decoder) Skip() error
- func (d *Decoder) Token() (Token, error)
- type Directive
- func (d Directive) Copy() Directive
- type Encoder
- func NewEncoder(w io.Writer) *Encoder
- func (enc *Encoder) Encode(v interface{}) error
- func (enc *Encoder) EncodeElement(v interface{}, start StartElement) error
- func (enc *Encoder) EncodeToken(t Token) error
- func (enc *Encoder) Flush() error
- func (enc *Encoder) Indent(prefix, indent string)
- type EndElement
- type Marshaler
- type MarshalerAttr
- type Name
- type ProcInst
- func (p ProcInst) Copy() ProcInst
- type StartElement
- func (e StartElement) Copy() StartElement
- func (e StartElement) End() EndElement
- type SyntaxError
- func (e *SyntaxError) Error() string
- type TagPathError
- func (e *TagPathError) Error() string
- type Token
- func CopyToken(t Token) Token
- type UnmarshalError
- func (e UnmarshalError) Error() string
- type Unmarshaler
- type UnmarshalerAttr
- type UnsupportedTypeError
- func (e *UnsupportedTypeError) Error() string
- Bugs
示例
Encoder MarshalIndent Unmarshal
包文件
marshal.go read.go typeinfo.go xml.go
常量
const (
// 适用于Marshal输出的通用XML头。
// 这不会自动添加到此包的任何输出中,
// 它是为了方便而提供的。
Header = `<?xml version="1.0" encoding="UTF-8"?>` + "\n"
)
变量
HTMLAutoClose 是应被视为自动关闭的一组 HTML 元素。
var HTMLAutoClose = htmlAutoClose
HTMLEntity 是包含标准 HTML 实体字符翻译的实体映射。
var HTMLEntity = htmlEntity
func Escape(查看源代码)
func Escape(w io.Writer, s []byte)
Escape 与 EscapeText 类似,但省略了错误返回值。它提供了与 Go 1.0 的向后兼容性。代码定位 Go 1.1 或更高版本应该使用 EscapeText。
func EscapeText(查看源代码)
func EscapeText(w io.Writer, s []byte) error
EscapeText 写入正确转义的纯文本数据的 XML 等价物。
func Marshal(查看源代码)
func Marshal(v interface{}) ([]byte, error)
Marshal 返回 v 的 XML 编码。
Marshal 通过封送每个元素来处理数组或片段。Marshal 通过编组指向的值处理指针,如果指针为零,则不写任何内容。Marshal 通过封送其包含的值来处理接口值,或者如果接口值为零,则不写任何内容。Marshal 通过编写一个或多个包含数据的 XML 元素来处理所有其他数据。
XML 元素的名称取自优先顺序:
- XMLName字段上的标记,如果数据是结构
- Name类型的XMLName字段的值
- 用于获取数据的struct字段的标记
- 用于获取数据的struct字段的名称
- 编组类型的名称
结构的 XML 元素包含每个结构导出字段的封送元素,但有以下例外:
- 省略了上述XMLName字段。
- 省略了带有“ - ”标记的字段。
- 带有“name,attr”标记的字段成为一个属性
XML元素中的给定名称。
- 带有标记“,attr”的字段成为带有的属性
XML元素中的字段名称。
- 带有标记“,chardata”的字段被写为字符数据,
不是作为XML元素。
- 带有标记“,cdata”的字段被写为字符数据
包含在一个或多个<![CDATA [...]]>标记中,而不是XML元素。
- 带有标记“,innerxml”的字段是逐字写入的,而不是主题
通常的编组程序。
- 带有标记“,comment”的字段被写为XML注释,而不是
遵循通常的编组程序。它不能包含
其中的“ - ”字符串。
- 省略了带有包含“omitempty”选项的标签的字段
如果字段值为空。空值为false,0,any
nil指针或接口值,以及任何数组,切片,映射或
长度为零的字符串。
- 处理匿名结构字段,就像它的字段一样
value是外部结构的一部分。
如果一个字段使用标签“a> b> c”,那么元素 c 将嵌套在父元素 a 和 b 中。名称相同的父对象旁边出现的字段将被包含在一个 XML 元素中。
以 MarshalIndent 为例。
如果要求编组频道,功能或地图,元帅将返回错误。
func MarshalIndent(查看源代码)
func MarshalIndent(v interface{}, prefix, indent string) ([]byte, error)
MarshalIndent 的工作方式与 Marshal 相同,但每个 XML 元素都以一个新的缩进行开始,该行以前缀开头,后跟一个或多个根据嵌套深度缩进的缩进副本。
示例
package main
import (
"encoding/xml"
"fmt"
"os"
)
func main() {
type Address struct {
City, State string
}
type Person struct {
XMLName xml.Name `xml:"person"`
Id int `xml:"id,attr"`
FirstName string `xml:"name>first"`
LastName string `xml:"name>last"`
Age int `xml:"age"`
Height float32 `xml:"height,omitempty"`
Married bool
Address
Comment string `xml:",comment"`
}
v := &Person{Id: 13, FirstName: "John", LastName: "Doe", Age: 42}
v.Comment = " Need more details. "
v.Address = Address{"Hanga Roa", "Easter Island"}
output, err := xml.MarshalIndent(v, " ", " ")
if err != nil {
fmt.Printf("error: %v\n", err)
}
os.Stdout.Write(output)
}
func Unmarshal(查看源代码)
func Unmarshal(data []byte, v interface{}) error
Unmarshal 解析 XML 编码的数据并将结果存储在v指向的值中,该值必须是任意的结构体,切片或字符串。丢弃不适合v的格式良好的数据。
由于 Unmarshal 使用反射包,因此它只能分配给导出(大写)字段。Unmarshal 使用区分大小写的比较来将 XML 元素名称与标记值和结构字段名称进行匹配。
Unmarshal 使用以下规则将 XML 元素映射到结构。在规则中,字段的标记引用与 struct 字段标记中的键 'xml' 关联的值(请参见上面的示例)。
*如果struct具有[]字节类型的字段或带标记的字符串
“,innerxml”,Unmarshal累积嵌套在其中的原始XML
该领域的元素。其余规则仍然适用。
*如果结构有一个名为XMLName的字段名称,
Unmarshal在该字段中记录元素名称。
*如果XMLName字段具有表单的关联标记
“name”或“namespace-URL name”,XML元素必须具有
给定的名称(以及可选的名称空间)或Unmarshal
返回错误。
*如果XML元素具有名称与a匹配的属性
struct field name,其关联标记包含“,attr”或
“name,attr”形式的struct field标签中的显式名称,
Unmarshal在该字段中记录属性值。
*如果XML元素的属性未由前一个处理
规则和struct有一个字段,其中包含相关的标记
“,any,attr”,Unmarshal在第一个中记录属性值
这样的领域。
*如果XML元素包含字符数据,那么该数据就是
累积在第一个具有标记“,chardata”的struct字段中。
struct字段可以有type [] byte或string。
如果没有这样的字段,则丢弃字符数据。
*如果XML元素包含注释,则会累积它们
第一个带有标记“,comment”的struct字段。结构
字段可以有[]字节或字符串。如果没有这样的话
字段,评论被丢弃。
*如果XML元素包含名称匹配的子元素
格式化为“a”或“a> b> c”的标记的前缀,unmarshal
将下降到XML结构中寻找带有的元素
给定名称,并将最内层元素映射到该结构
领域。以“>”开头的标签相当于一个开始
字段名称后跟“>”。
*如果XML元素包含名称匹配的子元素
struct字段的XMLName标记,struct字段没有
根据先前规则的显式名称标签,解组地图
该struct字段的子元素。
*如果XML元素包含名称与a匹配的子元素
没有任何模式标志的字段(“,attr”,“,chardata”等),Unmarshal
将子元素映射到该struct字段。
*如果XML元素包含未匹配任何子元素
以上规则和struct有一个标记为“,any”的字段,
unmarshal将子元素映射到该struct字段。
*处理匿名结构字段,就像它的字段一样
value是外部结构的一部分。
*带有标记“ - ”的结构字段永远不会被解组。
Unmarshal 将 XML 元素映射到字符串或 []byte,方法是将该元素的字符数据串联在字符串或 []byte 中。保存的 []byte 永远不会为零。
Unmarshal 通过将值保存在字符串或切片中将属性值映射到字符串或 []byte。
Unmarshal 通过将属性(包括其名称)保存在 Attr 中将属性值映射到 Attr。
Unmarshal 通过扩展切片的长度并将元素或属性映射到新创建的值来将 XML 元素或属性值映射到切片。
Unmarshal 通过将 XML 元素或属性值设置为由字符串表示的布尔值来映射 XML 元素或属性值。
Unmarshal 通过将字段设置为以十进制解释字符串值的结果将 XML 元素或属性值映射到整数或浮点字段。没有检查溢出。
Unmarshal 通过记录元素名称将 XML 元素映射到名称。
Unmarshal 通过将指针设置为新分配的值,然后将该元素映射到该值,将 XML 元素映射到指针。
缺少的元素或空属性值将被解组为零值。如果该字段是切片,则会将零值附加到该字段。否则,该字段将被设置为其零值。
示例
本示例演示了将 XML 摘录解编到具有某些预设字段的值中。请注意,Phone 字段未被修改,并且 XML <Company> 元素被忽略。此外,Groups 字段是考虑到其标签中提供的元素路径分配的。
package main
import (
"encoding/xml"
"fmt"
)
func main() {
type Email struct {
Where string `xml:"where,attr"`
Addr string
}
type Address struct {
City, State string
}
type Result struct {
XMLName xml.Name `xml:"Person"`
Name string `xml:"FullName"`
Phone string
Email []Email
Groups []string `xml:"Group>Value"`
Address
}
v := Result{Name: "none", Phone: "none"}
data := `
<Person>
<FullName>Grace R. Emlin</FullName>
<Company>Example Inc.</Company>
<Email where="home">
<Addr>gre@example.com</Addr>
</Email>
<Email where='work'>
<Addr>gre@work.com</Addr>
</Email>
<Group>
<Value>Friends</Value>
<Value>Squash</Value>
</Group>
<City>Hanga Roa</City>
<State>Easter Island</State>
</Person>
`
err := xml.Unmarshal([]byte(data), &v)
if err != nil {
fmt.Printf("error: %v", err)
return
}
fmt.Printf("XMLName: %#v\n", v.XMLName)
fmt.Printf("Name: %q\n", v.Name)
fmt.Printf("Phone: %q\n", v.Phone)
fmt.Printf("Email: %v\n", v.Email)
fmt.Printf("Groups: %v\n", v.Groups)
fmt.Printf("Address: %v\n", v.Address)
}
type Attr(查看源代码)
Attr 表示 XML 元素中的属性(Name = Value)。
type Attr struct {
Name Name
Value string
}
type CharData(查看源代码)
CharData表示XML字符数据(原始文本),其中XML转义序列已由它们表示的字符替换。
type CharData []byte
func (CharData) Copy(查看源代码)
func (c CharData) Copy() CharData
type Comment(查看源代码)
评论表示形式为 <!--comment--> 的 XML 注释。这些字节不包括 <!-- and --> 注释标记。
type Comment []byte
func (Comment) Copy(查看源代码)
func (c Comment) Copy() Comment
type Decoder(查看源代码)
解码器代表读取特定输入流的 XML 解析器。解析器假定它的输入是用 UTF-8 编码的。
type Decoder struct {
// 严格默认为true,强制执行要求
// XML规范。
// 如果设置为false,则解析器允许包含common的输入
// 错误:
// *如果元素缺少结束标记,则解析器会发明
// 必要时结束标记以保持令牌的返回值
// 适当平衡。
// *在属性值和字符数据中,未知或格式错误
// 字符实体(以&开头的序列)保持不变。
//
// 设置:
//
// d.Strict = false;
// d.AutoClose = HTMLAutoClose;
// d.Entity = HTMLEntity
//
// 创建一个可以处理典型HTML的解析器。
//
// 严格模式不强制要求XML名称空间TR。
// 特别是它不会拒绝使用未定义前缀的名称空间标记。
// 这些标签以未知前缀记录为名称空间URL。
Strict bool
// 当Strict == false时,AutoClose指示一组元素
// 无论如何,考虑在它们被打开后立即关闭
// 是否存在末端元素
AutoClose []string
// 实体可用于将非标准实体名称映射到字符串替换。
// 解析器的行为就像地图中存在这些标准映射一样,
// 无论实际的地图内容如何:
//
// "lt": "<",
// "gt": ">",
// "amp": "&",
// "apos": "'",
// "quot": `"`,
Entity map[string]string
// CharsetReader,如果是非nil,则定义要生成的函数
// charset-conversion readers,从提供的转换
// 非UTF-8字符集转换为UTF-8。 如果CharsetReader为零或
// 返回错误,解析因错误而停止。 其中一个
// CharsetReader的结果值必须是非零的。
CharsetReader func(charset string, input io.Reader) (io.Reader, error)
// 默认空间设置用于未修饰标签的默认命名空间,
// 好像整个XML流都包含在一个包含的元素中
// the attribute xmlns="DefaultSpace"。
DefaultSpace string
// 包含已过滤或未导出的字段
}
func NewDecoder(查看源代码)
func NewDecoder(r io.Reader) *Decoder
NewDecoder 从 r 中创建一个新的 XML 分析器。如果 r 没有实现 io.ByteReader,NewDecoder 会自行缓冲。
func (*Decoder) Decode(查看源代码)
func (d *Decoder) Decode(v interface{}) error
解码像 Unmarshal 一样工作,除了它读取解码器流以查找开始元素。
func (*Decoder) DecodeElement(查看源代码)
func (d *Decoder) DecodeElement(v interface{}, start *StartElement) error
DecodeElement 的工作方式与 Unmarshal 类似,只不过它需要一个指向开始 XML 元素的指针来解码为 v。当客户端读取一些原始 XML 令牌本身时,它也很有用,但也希望延迟 Unmarshal 的某些元素。
func (*Decoder) InputOffset(查看源代码)
func (d *Decoder) InputOffset() int64
InputOffset 返回当前解码器位置的输入流字节偏移量。偏移量给出了最近返回的标记的结束位置和下一个标记的开始位置。
func (*Decoder) RawToken(查看源代码)
func (d *Decoder) RawToken() (Token, error)
RawToken 与 Token 类似,但不验证开始和结束元素是否匹配,也不会将名称空间前缀转换为相应的 URL。
func (*Decoder) Skip(查看源代码)
func (d *Decoder) Skip() error
跳过读取标记,直到它消耗了与已经消耗的最近开始元素相匹配的结束元素。如果它遇到一个开始元素,它会重新出现,所以它可以用来跳过嵌套结构。如果找到匹配 start 元素的结束元素,则返回 nil;否则会返回描述问题的错误。
func (*Decoder) Token(查看源代码)
func (d *Decoder) Token() (Token, error)
令牌返回输入流中的下一个XML令牌。在输入流结束时,令牌返回 nil,io.EOF。
返回的标记数据中的字节片段指的是解析器的内部缓冲区,并且仅在下一次调用 Token 之前保持有效。要获取字节的副本,请调用 CopyToken 或标记的 Copy 方法。
令牌将自闭元素扩展为由连续调用返回的独立开始和结束元素。
令牌保证它返回的 StartElement 和 EndElement 令牌被正确嵌套和匹配:如果令牌在所有预期的结束元素之前遇到意外的结束元素或 EOF,它将返回一个错误。
Token 按照 http://www.w3.org/TR/REC-xml-names/ 所述实现 XML 名称空间。Token 中包含的每个 Name 结构都将空间设置为识别其名称空间的 URL。如果令牌遇到无法识别的名称空间前缀,它将使用前缀作为空格,而不是报告错误。
type Directive(查看源代码)
指令表示<!text>格式的 XML 指令。字节不包含 <! 和 > 标记。
type Directive []byte
func (Directive) Copy(查看源代码)
func (d Directive) Copy() Directive
type Encoder(查看源代码)
编码器将 XML 数据写入输出流。
type Encoder struct {
// 包含已过滤或未导出的字段
}
示例
package main
import (
"encoding/xml"
"fmt"
"os"
)
func main() {
type Address struct {
City, State string
}
type Person struct {
XMLName xml.Name `xml:"person"`
Id int `xml:"id,attr"`
FirstName string `xml:"name>first"`
LastName string `xml:"name>last"`
Age int `xml:"age"`
Height float32 `xml:"height,omitempty"`
Married bool
Address
Comment string `xml:",comment"`
}
v := &Person{Id: 13, FirstName: "John", LastName: "Doe", Age: 42}
v.Comment = " Need more details. "
v.Address = Address{"Hanga Roa", "Easter Island"}
enc := xml.NewEncoder(os.Stdout)
enc.Indent(" ", " ")
if err := enc.Encode(v); err != nil {
fmt.Printf("error: %v\n", err)
}
}
func NewEncoder(查看源代码)
func NewEncoder(w io.Writer) *Encoder
NewEncoder 返回一个写入 w 的新编码器。
func (*Encoder) Encode(查看源代码)
func (enc *Encoder) Encode(v interface{}) error
编码将 v 的 XML 编码写入流。
有关将 Go 值转换为 XML 的详细信息,请参阅 Marshal 的文档。
在返回之前编码调用Flush(刷新)。
func (*Encoder) EncodeElement(查看源代码)
func (enc *Encoder) EncodeElement(v interface{}, start StartElement) error
EncodeElement 将 v 的 XML 编码写入流,使用 start 作为编码中最外层的标记。
有关将 Go 值转换为 XML 的详细信息,请参阅 Marshal 的文档。
EncodeElement 在返回之前调用 Flush。
func (*Encoder) EncodeToken(查看源代码)
func (enc *Encoder) EncodeToken(t Token) error
EncodeToken 将给定的 XML 令牌写入流中。如果 StartElement 和 EndElement 标记没有正确匹配,它将返回一个错误。
EncodeToken 不会调用 Flush,因为它通常是 Encode 或 EncodeElement(或在这些操作期间调用的自定义 Marshaler 的MarshalXML)的较大操作的一部分,并且在完成时会调用 Flush。创建编码器然后直接调用 EncodeToken 而不使用 Encode 或 EncodeElement 的调用者在完成后需要调用 Flush,以确保将 XML 写入底层编写器。
EncodeToken 只允许将 Target 设置为“xml”的 ProcInst 作为流中的第一个标记。
func (*Encoder) Flush(查看源代码)
func (enc *Encoder) Flush() error
刷新将任何缓冲的 XML 刷新到底层写入器。有关何时需要的详细信息,请参阅 EncodeToken 文档。
func (*Encoder) Indent(查看源代码)
func (enc *Encoder) Indent(prefix, indent string)
缩进将编码器设置为生成 XML,其中每个元素都以新的缩进行开头,缩进行以前缀开头,后跟缩进的一个或多个副本(根据嵌套深度)。
type EndElement(查看源代码)
EndElement 表示一个 XML 结束元素。
type EndElement struct {
Name Name
}
type Marshaler(查看源代码)
Marshaler 是由对象实现的接口,可以将自己编组为有效的 XML 元素。
MarshalXML 将接收器编码为零个或多个 XML 元素。按照惯例,数组或片通常被编码为一系列元素,每个条目一个。不需要使用 start 作为元素标记,但这样做可以使 Unmarshal 将 XML 元素与正确的 struct 字段进行匹配。一种常见的实现策略是使用与所需 XML 相对应的布局构造一个单独的值,然后使用 e.EncodeElement 对其进行编码。另一种常见策略是对 e.EncodeToken 使用重复调用来一次生成一个令牌的 XML 输出。编码令牌的序列必须组成零个或多个有效的 XML 元素。
type Marshaler interface {
MarshalXML(e *Encoder, start StartElement) error
}
type MarshalerAttr(查看源代码)
MarshalerAttr 是由对象实现的接口,可以将自己编组为有效的 XML 属性。
MarshalXMLAttr 返回一个 XML 属性和接收者的编码值。使用 name 作为属性名称不是必需的,但这样做将使 Unmarshal 能够将该属性与正确的 struct 字段进行匹配。如果 MarshalXMLAttr 返回零属性 Attr{},则不会在输出中生成任何属性。MarshalXMLAttr 仅用于字段标签中具有“attr”选项的结构字段。
type MarshalerAttr interface {
MarshalXMLAttr(name Name) (Attr, error)
}
type Name(查看源代码)
名称表示用名称空间标识符(Space)注释的XML名称(Local)。在由 Decoder.Token 返回的令牌中,空间标识符是作为规范 URL 提供的,而不是被解析文档中使用的短前缀。
type Name struct {
Space, Local string
}
type ProcInst(查看源代码)
ProcInst表示<?target inst?>形式的XML处理指令
type ProcInst struct {
Target string
Inst []byte
}
func (ProcInst) Copy(查看源代码)
func (p ProcInst) Copy() ProcInst
type StartElement(查看源代码)
StartElement 表示 XML 起始元素。
type StartElement struct {
Name Name
Attr []Attr
}
func (StartElement) Copy(查看源代码)
func (e StartElement) Copy() StartElement
func (StartElement) End(查看源代码)
func (e StartElement) End() EndElement
End 返回相应的 XML 结束元素。
type SyntaxError(查看源代码)
SyntaxError 表示 XML 输入流中的语法错误。
type SyntaxError struct {
Msg string
Line int
}
func (*SyntaxError) Error(查看源代码)
func (e *SyntaxError) Error() string
type TagPathError(查看源代码)
TagPathError 表示由于使用带有冲突路径的字段标记而导致解组过程中出现错误。
type TagPathError struct {
Struct reflect.Type
Field1, Tag1 string
Field2, Tag2 string
}
func (*TagPathError) Error(查看源代码)
func (e *TagPathError) Error() string
type Token(查看源代码)
令牌是一个持有令牌类型之一的接口:StartElement,EndElement,CharData,Comment,ProcInst或Directive。
type Token interface{}
func CopyToken(查看源代码)
func CopyToken(t Token) Token
CopyToken 返回一个令牌的副本。
type UnmarshalError(查看源代码)
UnmarshalError 表示解组过程中的错误。
type UnmarshalError string
func (UnmarshalError) Error(查看源代码)
func (e UnmarshalError) Error() string
type Unmarshaler(查看源代码)
Unmarshaler 是可以解组自己的 XML 元素描述的对象实现的接口。
UnmarshalXML 解码从给定的开始元素开始的单个 XML 元素。如果它返回一个错误,对 Unmarshal 的外部调用将停止并返回该错误。UnmarshalXML 必须使用一个 XML 元素。一种常见的实现策略是使用 d.DecodeElement 解组为一个单独的值,其布局与预期的 XML 匹配,然后将该值中的数据复制到接收器中。另一个常用策略是使用 d.Token 一次处理 XML 对象的一个令牌。UnmarshalXML 不能使用 d.RawToken。
type Unmarshaler interface {
UnmarshalXML(d *Decoder, start StartElement) error
}
type UnmarshalerAttr(查看源代码)
UnmarshalerAttr 是由可以解组自己的 XML 属性描述的对象实现的接口。
UnmarshalXMLAttr 解码单个 XML 属性。如果它返回一个错误,对Unmarshal 的外部调用将停止并返回该错误。UnmarshalXMLAttr 仅用于字段标签中具有“attr”选项的结构字段。
type UnmarshalerAttr interface {
UnmarshalXMLAttr(attr Attr) error
}
type UnsupportedTypeError(查看源代码)
MarshalXMLError 在 Marshal 遇到无法转换为 XML 的类型时返回。
type UnsupportedTypeError struct {
Type reflect.Type
}
func (*UnsupportedTypeError) Error(查看源代码)
func (e *UnsupportedTypeError) Error() string
Bugs
- ☞XML 元素和数据结构之间的映射本质上是有缺陷的:XML元素是匿名值的依赖于顺序的集合,而数据结构是与命令值无关的顺序无关集合。查看包json以获得更适合数据结构的文本表示。
编码 | encoding相关
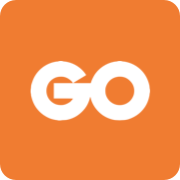
Go 是一种编译型语言,它结合了解释型语言的游刃有余,动态类型语言的开发效率,以及静态类型的安全性。它也打算成为现代的,支持网络与多核计算的语言。要满足这些目标,需要解决一些语言上的问题:一个富有表达能力但轻量级的类型系统,并发与垃圾回收机制,严格的依赖规范等等。这些无法通过库或工具解决好,因此Go也就应运而生了。
主页 | https://golang.org/ |
源码 | https://go.googlesource.com/go |
发布版本 | 1.9.2 |