React native参考手册
APIs
StyleSheet
StyleSheet是一个类似于CSS StyleSheets的抽象
创建一个新的样式表:
const styles = StyleSheet.create({
container: {
borderRadius: 4,
borderWidth: 0.5,
borderColor: '#d6d7da',
},
title: {
fontSize: 19,
fontWeight: 'bold',
},
activeTitle: {
color: 'red',
},
});
使用样式表:
<View style={styles.container}>
<Text style={[styles.title, this.props.isActive && styles.activeTitle]} />
</View>
代码质量:
- 通过将样式从渲染函数中移走,可以让代码更易于理解。
- 命名样式是为渲染函数中的低级组件添加含义的好方法。
性能:
- 从样式对象中创建样式表可以通过ID引用它,而不是每次创建新的样式对象。
- 它也允许只通过桥发送一次风格。所有后续使用都将引用一个id(尚未实现)。
方法
静态setStyleAttributePreprocessor(属性,进程)
警告:实验。突然发生的变化可能会发生很多并且不会可靠地宣布。整个事情可能会被删除,谁知道?使用风险自负。
设置用于预处理样式属性值的函数。这用于内部处理颜色和变换值。除非你真的知道自己在做什么并且已经用尽了其他选项,否则你不应该使用它。
静态创建(obj)
从给定的对象创建一个StyleSheet样式引用。
属性
hairlineWidth: CallExpression
这被定义为平台上细线的宽度。它可以用作边界或两个元素之间的分界线的厚度。例:
{
borderBottomColor: '#bbb',
borderBottomWidth: StyleSheet.hairlineWidth
}
这个常量将总是像素的一个整数(所以它定义的一条线看起来很清晰),并且会尝试匹配底层平台上细线的标准宽度。但是,您不应该依赖它的大小不变,因为在不同的平台和屏幕密度下,其值可能会有不同的计算方式。
如果您的模拟器缩小了比例,则发线宽度的线条可能不可见。
absoluteFill: CallExpression
一个非常常见的模式是创建绝对位置和零位置的覆盖图,因此absoluteFill
可以方便使用并减少这些重复样式的重复。
absoluteFillObject: ObjectExpression
有时候你可能想要,absoluteFill
但有一些调整 - absoluteFillObject
可以用来创建一个自定义的条目StyleSheet
,例如:
const styles = StyleSheet.create({wrapper:{... StyleSheet.absoluteFillObject,top:10,backgroundColor:'transparent',},});
flatten: CallExpression
将一组样式对象平铺到一个聚合样式对象中。或者,此方法可用于查找由StyleSheet.register返回的ID。
注意:谨慎使用此操作会导致优化。ID通常可以通过网桥和内存进行优化。直接引用样式对象会剥夺您这些优化。
例:
const styles = StyleSheet.create({
listItem: {
flex: 1,
fontSize: 16,
color: 'white'
},
selectedListItem: {
color: 'green'
}
});
StyleSheet.flatten([styles.listItem, styles.selectedListItem])
// returns { flex: 1, fontSize: 16, color: 'green' }
替代用途:
StyleSheet.flatten(styles.listItem);
// return { flex: 1, fontSize: 16, color: 'white' }
// Simply styles.listItem would return its ID (number)
此方法在内部用于StyleSheetRegistry.getStyleByID(style)
解析由ID表示的样式对象。因此,一组样式对象(StyleSheet.create的实例)被单独解析为它们各自的对象,并合并为一个然后返回。这也解释了替代用途。
APIs相关
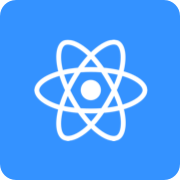
React Native 是一个 JavaScript 的框架,用来撰写实时的、可原生呈现 iOS 和 Android 的应用。
主页 | https://facebook.github.io/react-native/ |
源码 | https://github.com/facebook/react-native |
发布版本 | 0.49 |